6.10.94-stable
GreyCat Modules
- Greycat code is organized by modules which are basically files with the .gcl extension
- The main module of any greycat project should be named project.gcl (At the top level directory of the project)
- A module can contain several global variables, functions, enums and type definitions
- For example here is a bank.gcl module:
// Global module variables
var countries_by_name: nodeIndex<String, node<Country>>; //Global index to index all countries
// Global module functions
fn printStats() {
// Some global module function called printStats
}
//Several Enum and Type definitions
enum TransactionType {
online("online");
transfer("transfer");
}
type Country {
name: String;
banks: nodeIndex<String, node<Bank>>;
}
type Bank {
name: String;
location: geo;
}
Greycat naming convention
Item | Convention | Example |
---|---|---|
Modules | snake_case | smart_city.gcl |
Types / Class / Enum | UpperCamelCase | enum TransactionChannel |
Primitive types | camelCase | nodeTime, nodeIndex, int |
Functions | camelCase / snake_case * | fn getCities() |
Variables / Attributes | camelCase / snake_case * | var cities_by_name: nodeIndex; |
- For functions and attributes we leave the freedom of camelCase/snake_case according to readability
- Usually index names are more readable in snake_case: city_by_location, city_by_name
- Simple attributes or variable names are usually in camelCase: firstName, primaryContract
Greycat project structure
- By convention, we organize greycat modules in the following directory structure:
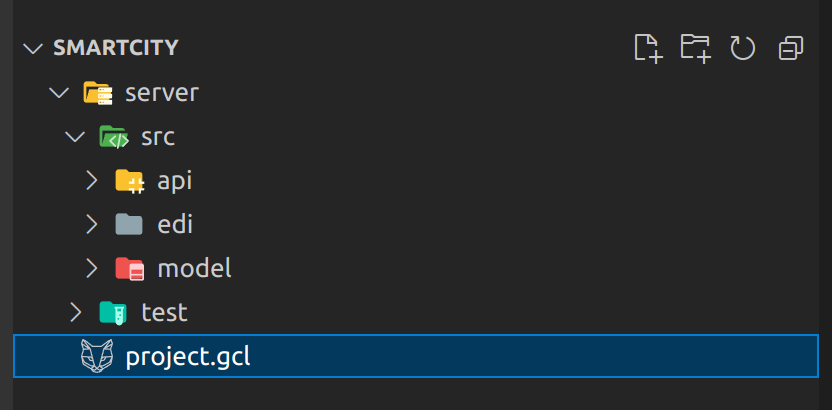
- At the top level there is the project.gcl file and server folder.
- /server folder represents the backend
- /server/src contains the backend source code:
- /server/src/model : core data model for the domain
- /server/src/api : application programming interface (for frontend - backend communication)
- /server/src/edi : electronic data interface (for data importer/exporter)
- /server/test contains the backend testing code