7.1.483-stable
Project
Versioning
To manage you greycat version on a per project specify the library std version in your project.gcl
// project.gcl
@library("std", "7.0.1304-dev");
greycat install
This will create two directories
lib : will contain the libraries bin : will contain the greycat executable, from which you should execute your greycat application
GreyCat Modules
- GreyCat code is organized by modules which are basically files with the .gcl extension
- The main module of any GreyCat project must be named project.gcl (At the top level directory of the project)
- A module can contain several global variables, functions, enums and type definitions
For example here is a bank.gcl module:
// Global module variables
var countries_by_name: nodeIndex<String, node<Country>>; // Global index to index all countries
// Global module functions
fn printStats() {
// Some global module function called printStats
}
// Several Enum and Type definitions
enum TransactionType {
online("online");
transfer("transfer");
}
type Country {
name: String;
banks: nodeIndex<String, node<Bank>>;
}
type Bank {
name: String;
location: geo;
}
All content from a module is automatically accessible in the whole project
New module called other.gcl
fn otherStuff() {
// function defined in bank.gcl
printStats();
// type defined in bank.gcl
var bank = Bank {
name: "BGL"
location: 49.613764lat_6lat_6.127357lnglng,
};
// var defined in bank.gcl
var country = countries_by_name.get("France");
}
GreyCat project structure
- By convention, we organize GreyCat modules in the following directory structure:
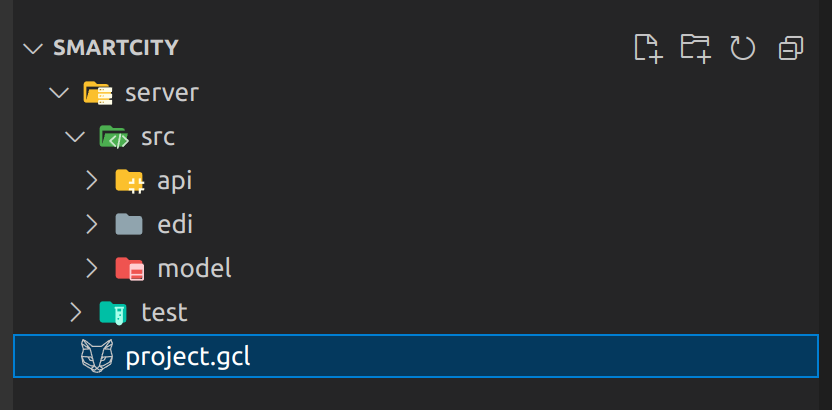
- At the top level there is the project.gcl file and server folder.
- /server folder represents the backend
- /server/src contains the backend source code:
- /server/src/model : core data model for the domain
- /server/src/api : application programming interface (for frontend - backend communication)
- /server/src/edi : electronic data interface (for data importer/exporter)
- /server/test contains the backend testing code